728x90
반응형
목차
1.주제
2.크롤링
2.1.필요한 패키지 임포트
2.2.
2.3.KBO 사이트 구조 확인하기
2.4.24 시즌 관중수 크롤링
2.5.23 시즌 관중 수 크롤링
2.6.22 시즌 관중 수 크롤링
주제
저번에 KBO 공식 사이트에서 2024 시즌 관중 수 크롤링을 해 보았습니다.
24시즌 KBO 관중 수 시각화
시각화 주제2024 KBO 리그가 10개 구단 체재 출범 이후 최다 매진 경기 신기록을 세울 정도로 많은 팬분들이 야구장을 향하고 있습니다. 올해 프로야구의 열기는 개막전 모든 경기가 매진되면서
bbdiary03.tistory.com
이번에는 KBO 관중 수 중 삼성 라이온즈 관중 수만 크롤링해보겠습니다.
크롤링
필요한 패키지 임포트
import pandas as pd
import matplotlib.pyplot as plt
import matplotlib as mlp
import seaborn as sns
import requests
from bs4 import BeautifulSoup
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.support.ui import Select
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
from webdriver_manager.chrome import ChromeDriverManager
import time
KBO 사이트 구조 확인하기
KBO 사이트의 구조를 확인해 어디서 삼성 라이온즈로 설정을 해야 할지 확인합니다.
%matplotlib inline
# 한글 폰트 설정
plt.rcParams['font.family'] = 'NanumGothic'
req = requests.get('https://www.koreabaseball.com/Record/Crowd/GraphDaily.aspx')
soup = BeautifulSoup(req.text, 'html.parser')
soup
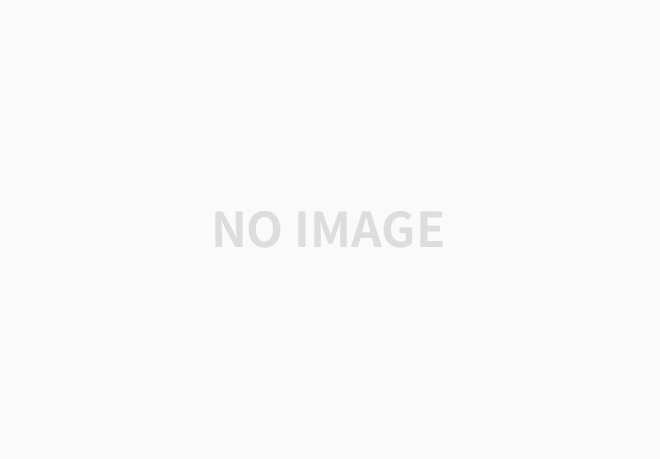
시즌, 팀별로 어떤 ID로 구정 되어있는지 찾아주고 크롤링을 해보겠습니다.
24 시즌 관중수 크롤링
def set_webdriver():
# ChromeDriverManager를 통해 드라이버 경로를 자동으로 설정합니다.
driver_path = ChromeDriverManager().install()
options = webdriver.ChromeOptions()
options.add_argument('--headless') # GUI 없는 모드
options.add_argument('--no-sandbox')
options.add_argument('--disable-dev-shm-usage')
driver = webdriver.Chrome(executable_path=driver_path, options=options)
return driver
# Selenium WebDriver 설정
driver = set_webdriver()
url = "https://www.koreabaseball.com/Record/Crowd/GraphDaily.aspx"
driver.get(url)
# 페이지가 로드될 때까지 잠시 기다립니다.
wait = WebDriverWait(driver, 10)
# 시즌, 월, 팀 선택 드롭다운을 기다립니다.
season_select = wait.until(EC.presence_of_element_located((By.ID,
"cphContents_cphContents_cphContents_ddlSeason")))
month_select = Select(driver.find_element(By.ID,
"cphContents_cphContents_cphContents_ddlMonth"))
team_select = Select(driver.find_element(By.ID,
"cphContents_cphContents_cphContents_ddlTeam"))
# 시즌을 2024로 선택
season_select = Select(season_select)
season_select.select_by_value("2024")
# 월을 0으로 선택 (전체)
month_select.select_by_value("0")
# 팀을 삼성으로 선택
team_select.select_by_value("SS")
# 검색 버튼 클릭
search_button = driver.find_element(By.ID, "cphContents_cphContents_cphContents_btnSearch")
search_button.click()
# 테이블이 로드될 때까지 잠시 기다립니다.
time.sleep(5)
# class 이름이 tData인 table을 가져옵니다
tdata = driver.find_element(By.CLASS_NAME, "tData")
rows = tdata.find_elements(By.TAG_NAME, "tr")
# 데이터 저장
data = []
for row in rows:
cells = row.find_elements(By.TAG_NAME, "td")
if len(cells) > 0:
data.append([cell.text for cell in cells])
# DataFrame으로 변환
columns = ["날짜", "요일", "홈", "방문", "구장", "관중수"]
df = pd.DataFrame(data, columns=columns)
# DataFrame 출력
print(df)
# 드라이버 종료
driver.quit()
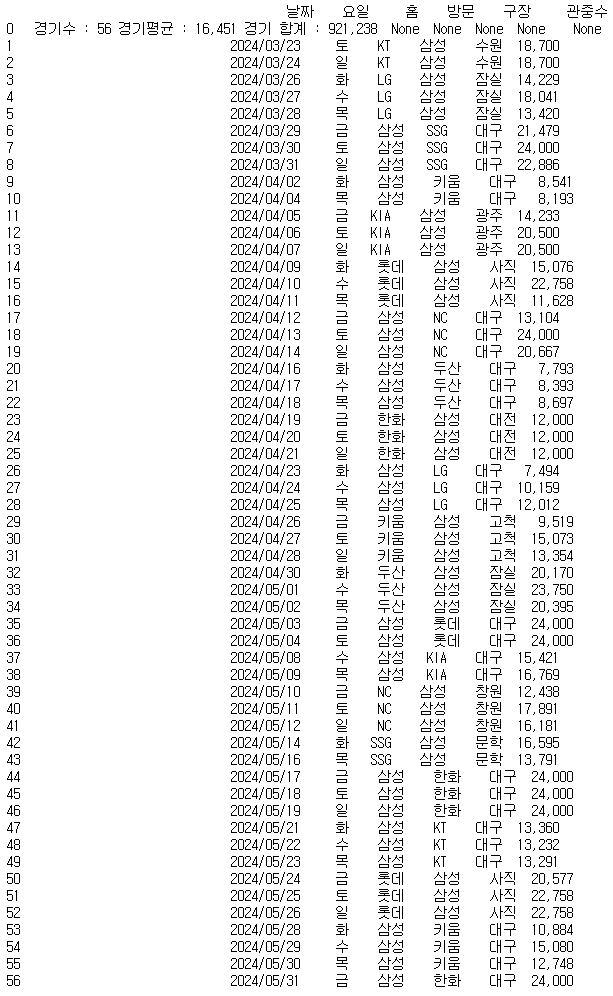
5/31일까지 총 56경기를 크롤링했고 평균 16,451명의 관중이 온 것을 알 수 있습니다.
23 시즌 관중 수 크롤링
24 시즌 크롤링을 한 것처럼 시즌만 23 시즌으로 바꿔주면 됩니다.
# 시즌을 2023로 선택
season_select = Select(season_select)
season_select.select_by_value("2023")
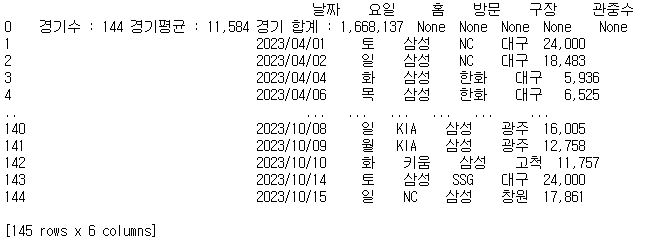
총 144경기를 했고 평균 관중은 11,584로 24 시즌보다는 적은 것을 알 수 있습니다.
22 시즌 관중 수 크롤링
22 시즌도 23 시즌과 마찬가지로 시즌만 22 시즌으로 바꿔주었습니다.
# 시즌을 2023로 선택
season_select = Select(season_select)
season_select.select_by_value("2022")
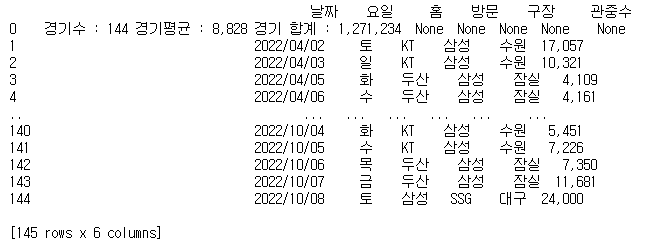
총 144경기를 했고 평균 관중은 8828명으로 다른 두 시즌보다 적은 것을 알 수 있습니다.
728x90
반응형
'야구 분석 > Python' 카테고리의 다른 글
삼성 라이온즈 성적 크롤링하기 (1) | 2024.06.09 |
---|---|
삼성 라이온즈 관중 수 시각화 (1) | 2024.06.05 |
24시즌 KBO 관중 수 시각화 (0) | 2024.05.29 |
타율이 높은 선수는 홈런도 많이 칠까? 2023 시즌 데이터로 본 상관관계 (0) | 2024.05.25 |
히트 스프레이 차트에 야구장 사진 넣어보기 (0) | 2024.05.22 |